WCAG 2.1 Checklist with Code Examples
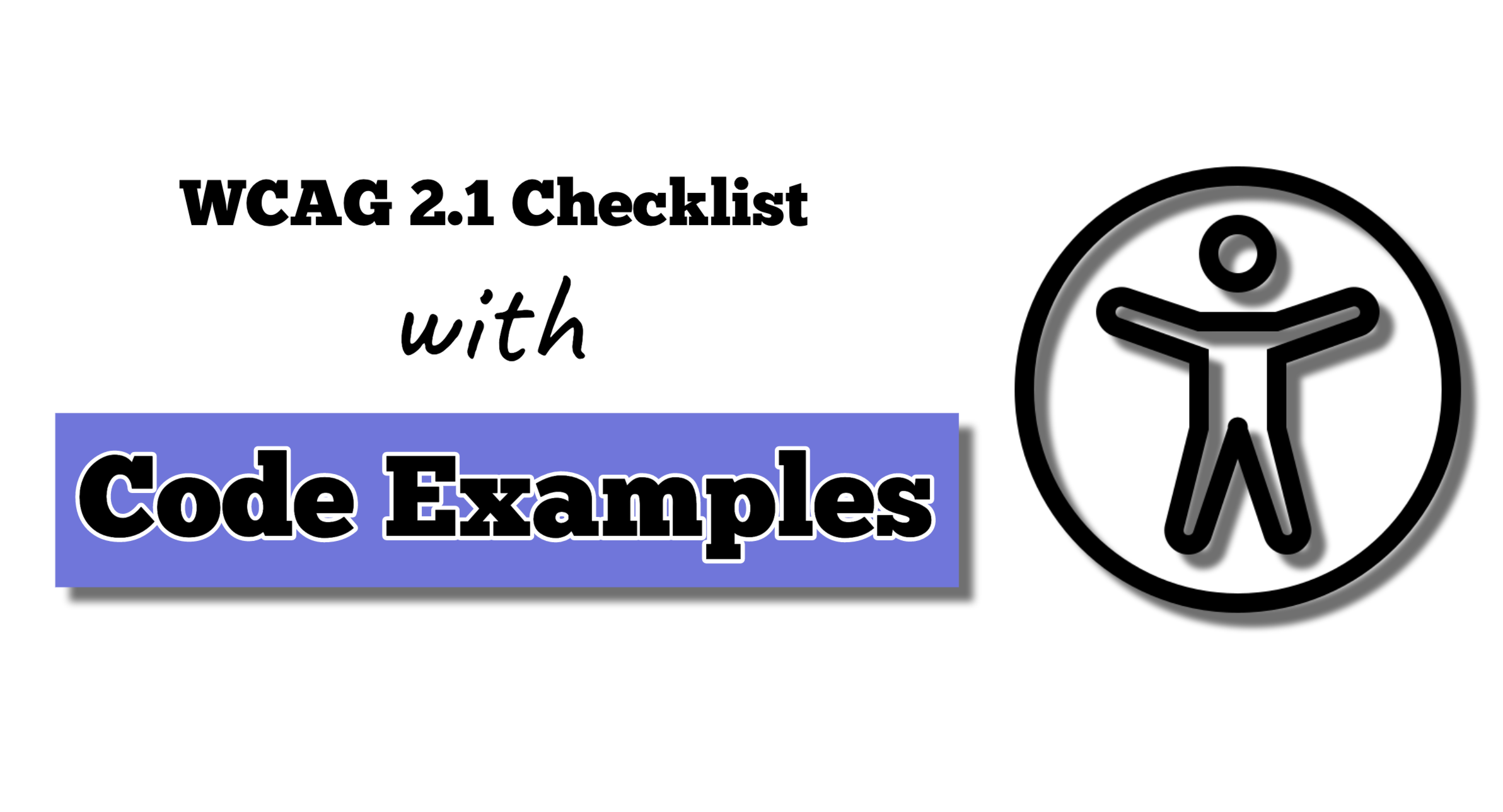
The WCAG checklist is a list of criteria that ensure web content and websites are accessible for all users.
This article will cover several WCAG guidelines with useful code snippets for each guideline.
WCAG 2.1 LEVEL A CHECKPOINTS
Principle 1: Perceivable
Information and user interface components must be presentable to users in ways they can perceive.
1.1. Text Alternatives
All non-text content such as images, icons, charts, etc must have alternative text that describes the content.
No alt attribute has been added to this image.
//bad code <img src="logo.png" />
An ait attribute has been added.
//good code <img src="logo.png" alt="company's logo" />
1.3.1 Info and Relationships
Information, structure, and relationships conveyed through presentation can be programmatically determined or are available in text.
A good example is adding proper semantic meaning and emphasis to text content.
Styling the text to appear bold may work for sighted users. However, screen readers will be unable to emphasize the text for visually impaired users.
//bad code <p>Get out of bed <span>now</span>!</p> span { font-weight: bold; }
Using the appropriate elements gives semantic meaning to the text and fixes this issue.
//good code Get out of bed <em>now</em>!
Principle 2: Operable
User interface components and navigation must be operable.
2.1.1 Keyboard
All functionality should be operable with a keyboard.
This div can be styled to appear like a button. However, it will not have semantic meaning or keyboard focus.
//bad code <div class="button">submit</div>
A simple solution is to use a button element.
//good code <button>submit</button>
If for some reason a div has to be used, adding a role and tabindex will make it keyboard operable.
<div role="button" tabindex="0">Close</div>
2.4.2 Page titled
Titles of the page should describe the topic or purpose.
The title of the page is not clear from this heading.
//bad code <h1>a website's page</h1>
The title of the page is clear from this heading.
//good code <h1>About Us</h1>
2.4.4 Link Purpose (in context)
The target or purpose of the link must be clear by the link text alone, its associated content, or its surrounding content.
The text does not properly describe the purpose of the link.
//bad code <a href="google.com">this is a link to a webpage</a>
The text clearly describes the purpose of the link.
//good code <a href="google.com">Go to Google</a>
2.5.3 Label in Name
For user interface components with labels that include text or images of text, the name contains the text that is presented visually.
A speech input user would say ‘buy’, but the speech software won’t know what item to add.
//bad code <button>buy</button>
To solve this issue, make the label and the accessible name the same.
//good code <button aria-label="buy shoes">buy shoes</button>
Principle 3: Understandable
Information and the operation of the user interface must be understandable.
3.1.1 Language of Page
Programmatically define the primary language of each page.
The lang attribute sets the language for a page.
<html lang="en"></html>
3.3.2 Labels or Instructions
Form inputs should have labels associated with them.
Form fields without labels associated with them are inaccessible.
//bad code <input name="userEmail" type="email" />
Associating form fields with labels gives users a larger clickable area. Labels also help users to fill in the correct information in the form field.
//good code <label for="email">Enter your email address</label> <input id="email" name="userEmail" type="email" />
Principle 4: Robust
Content must be robust enough that it can be interpreted by a wide variety of user agents, including assistive technologies.
4.1.1 Parsing
Make sure that the following are taken care of in the markup
– Elements should have unique IDs.
The IDs are similar; this is wrong.
//bad code <button id="button">submit</button> <button id="button">login</button>
The IDs are distinct; this is correct.
//good code <button id="submit">submit</button> <button id="login">login</button>
– Elements have proper opening and closing tags.
There is no closing tag.
//bad code <button>submit </button>
There is a closing tag.
//good code <button>submit</button>
– Elements are properly nested.
Block-level elements should not be nested in inline elements.
//bad code <p> <div>i am a block level element nested in an inline element. This is wrong</div> </p>
Inline elements can be nested in block-level elements.
//good code <div> <p>i am an inline element nested in a block level element. This is right.</p> </div>
4.1.2 Name, Role, Value
All interactive elements on a page should have a name and role, state. and value where applicable.
An image of a button was used instead of a button element.
//bad code <img src="button.png" alt="click the button" />
A button element was used.
//good code <input name="form submission button" type="submit" value="submit the form" />
WCAG 2.1 LEVEL AA CHECKPOINTS
Principle 1: Perceivable
1.3.4 Orientation
Content adapts to different screen sizes and display orientation.
By setting the width of the card to 500px, it will overflow on mobile phones and small screens.
//bad code .card { width: 500px; }
Changing the height to 500px with media queries ensures that the content adapts.
//good code .card { width: 100%; } @media screen and (min-width: 768px) { .card { width: 500px; } }
1.3.5 Identify Input Purpose
The purpose of input fields should be programmatically determinable.
The type attribute was not added to the input element.
//bad code <input name="userEmail" type="text" placeholder="enter your email address" />
Setting the type attribute to `email` helps assistive technologies know what type of data is required. This enables them to automatically fill out the form for users.
//good code <input name="userEmail" type="email" placeholder="enter your email address" />
1.4.4 Resize text
Text content should remain readable even when users zoom up to 200%.
With these static styles, when a user zooms in, the static sizing will affect the readability of the text.
//bad code .card-static-units { height: 200px; width: 400px; }
Using scalable sizing units fixes this issue.
//good code .card-dynamic-units { height: 12.5rem; width: 25rem; }
1.4.5 Images of text
Use real text as much as possible instead of images of text.
Screen readers will be unable to read text on images.
//bad code <img src="page heading" />
Therefore, text content must be presented in a proper and accessible format.
//good code</pre> <h1>Page Heading</h1>
1.4.10. Reflow
Content can be presented without loss of information or functionality, and without requiring
scrolling in two dimensions.
This style will cause horizontal scroll on small screens.
//bad code .card { width: 500px; }
Using responsive CSS fixes the issue.
//good code .card { width: 100%; } @media screen and (min-width: 768px) { .card { width: 500px; } }
Principle 2: Operable
2.4.6 Headings and Labels
Headings and labels should be descriptive.
A bad example of a descriptive heading.
//bad code <h1>Intro</h1>
A good example of a descriptive heading.
//good code <h1>Introduction to Accessibility</h1>
A bad example of a descriptive label.
//bad code <label>fill form</label> <input type="text" />
A good example of a descriptive label.
//good code <label>Name</label> <input name="name" type="text" />
2.4.7 Focus Visible
Provide a clearly visible focus indicator for all the interactive elements.
When the link is in focus, it will have a red border on it.
a:focus{ border: 1px solid red }
Principle 3: Understandable
3.1.2 Language of parts
If any text or element on the web page has a different language than the primary language, programmatically define the language for that content.
<html lang="en"> <body> <p lang="fr">I have a different language, French!</p> </body> </html>
Leave a Reply